🔴 Flutter google login with firebase authentication - devhubspot
Firebase is a product of Google that helps developers to build, manage, and grow their apps easily. It helps developers to build their apps faster and in a more secure way. Firebase Authentication provides backend services, easy-to-use SDKs, and ready-made UI libraries to authenticate users to your app. It supports authentication using passwords, phone numbers, and popular federated identity providers like Google, Facebook, Twitter, and more.
In this article, I will show how to set up a Flutter app and implement Google Sign-In using Firebase authentication.
Project Overview
The app layouts will be simple, consisting of just two screens. The initial screen will be a sign-in screen (where users can sign in using their Google account), and the next screen will be the user info screen (which will display some of the user information retrieved from one’s Google account) with a button for signing out.
Getting started is simple, follow the steps:
- Go to https://firebase.google.com/
- Click Get started
- Register your app
- A small advice, always fill in the SHA certificate fingerprint, even though it says optional

5. Go to
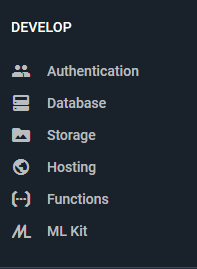
Click authentication and enable the Google sign-in (as we are trying for Google first)….
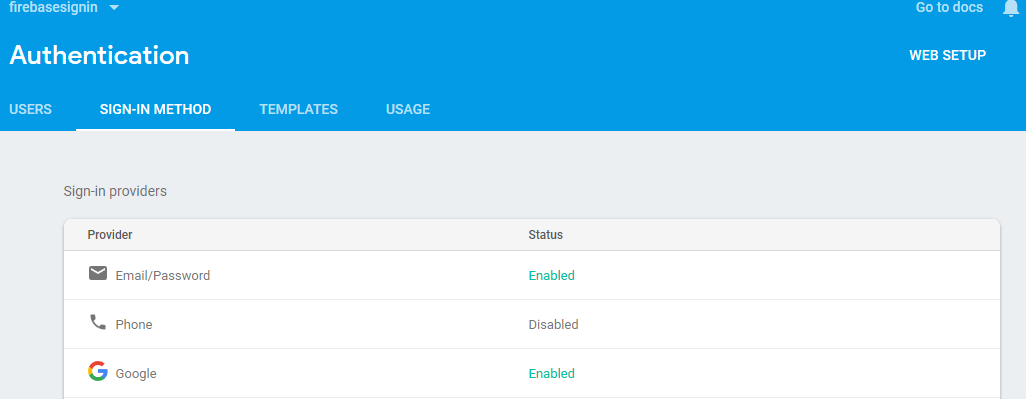
All setup, now programming part...
On click of Sign in with Google, the following function is called :
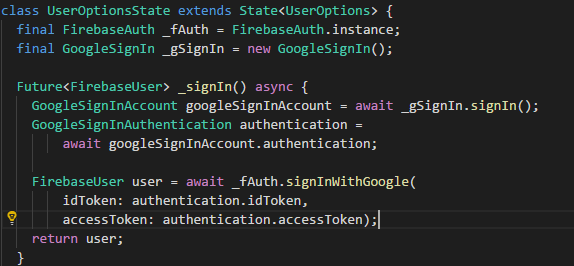
On click of Sign out,
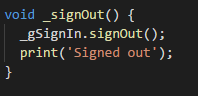
Create a new Flutter project
Open Terminal or use the terminal in your code editor. Navigate to the folder where you want to create the project, and use the following command:
flutter create flutterfire_samples
Then open the project using your favorite code editor. To open it with VS Code, you can use:
code flutterfire_samples
Flutter 2.0 has support for null safety in the stable channel, but in order to use it inside the app, you have to run a command to migrate the project to null safety.
Before running the migration command, check if all your current project dependencies support null safety by using:
dart pub outdated --mode=null-safety
Then, run the following command to migrate:
dart migrate
You can follow the migration guide here.
Plugins
The plugins needed for this project are:
- firebase_core: for initializing Firebase
- firebase_auth: for implementing Firebase authentication
- google_sign_in: to use Google Sign-In
You will need to include the
firebase_core
plugin for using any other Firebase-related plugins, as it is used for initializing theFirebaseApp()
.
You can import the packages to your Flutter project by adding them to your pubspec.yaml
file:
firebase_core: ^1.0.1
firebase_auth: ^1.0.1
google_sign_in: ^5.0.0
Save it to run flutter packages get
.
Assets
You will need two images while building the UI of this sample app.
Create a new folder called assets
in your project directory, and insert the two images (google_logo.png
and firebase_logo.png
) that you downloaded.
Now, import the assets
folder in your pubspec.yaml
file.
Here’s how our main.dart file would look like-
import 'package:flutter/material.dart'; import 'package:google_signin/pages/login_page.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Google SignIn', theme: ThemeData( primarySwatch: Colors.blue, ), home: LoginPage(), debugShowCheckedModeBanner: false, ); } }
Here’s the code for our login screen-
import 'package:flutter/material.dart'; import 'package:font_awesome_flutter/font_awesome_flutter.dart'; import 'package:google_sign_in/google_sign_in.dart'; import 'package:google_signin/pages/home_page.dart'; GoogleSignIn _googleSignIn = GoogleSignIn( scopes: <String>[ 'email', ], ); class LoginPage extends StatefulWidget { @override _LoginPageState createState() => _LoginPageState(); } class _LoginPageState extends State<LoginPage> { @override void initState() { super.initState(); _googleSignIn.onCurrentUserChanged.listen((GoogleSignInAccount account) { if (account != null) { Navigator.pushReplacement( context, MaterialPageRoute( builder: (context) => HomePage(), ), ); } }); _googleSignIn.signInSilently(); } @override Widget build(BuildContext context) { return SafeArea( child: Scaffold( body: Center( child: RaisedButton( shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(30), ), color: Colors.amber, child: Padding( padding: EdgeInsets.all(8), child: Row( mainAxisSize: MainAxisSize.min, children: <Widget>[ FaIcon( FontAwesomeIcons.google, ), SizedBox( width: 10, ), Text( 'Google', style: TextStyle( fontSize: 24, fontWeight: FontWeight.w800, ), ) ], ), ), onPressed: () { _handleSignIn(); }, ), ), ), ); } Future<void> _handleSignIn() async { try { await _googleSignIn.signIn(); } catch (error) { print(error); } } }
Here’s the code for our home screen-
import 'package:flutter/material.dart'; import 'package:font_awesome_flutter/font_awesome_flutter.dart'; import 'package:google_sign_in/google_sign_in.dart'; class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { GoogleSignIn _googleSignIn = GoogleSignIn( scopes: <String>[ 'email', ], ); GoogleSignInAccount user; @override void initState() { super.initState(); _googleSignIn.onCurrentUserChanged.listen((GoogleSignInAccount account) { if (account != null) { setState(() { user = account; }); } }); _googleSignIn.signInSilently(); } @override Widget build(BuildContext context) { return SafeArea( child: Scaffold( body: Padding( padding: const EdgeInsets.all(20), child: user != null ? Column( crossAxisAlignment: CrossAxisAlignment.start, children: <Widget>[ appBar(), SizedBox( height: 30, ), Text( 'Hello, ${user.displayName}!', style: TextStyle( fontSize: 30, color: Colors.grey[700], fontWeight: FontWeight.w600, ), ) ], ) : Container(), ), ), ); } Widget appBar() { return Row( mainAxisAlignment: MainAxisAlignment.spaceBetween, children: <Widget>[ FaIcon( FontAwesomeIcons.google, color: Colors.amber, size: 30, ), Row( mainAxisSize: MainAxisSize.min, children: <Widget>[ Stack( children: <Widget>[ ClipRRect( borderRadius: BorderRadius.all( Radius.circular(30), ), child: Image.network( user.photoUrl, height: 50, width: 50, ), ), Container( margin: EdgeInsets.only(left: 35), width: 13, height: 13, child: ClipRRect( borderRadius: BorderRadius.all(Radius.circular(10)), child: Container( color: Colors.green[300], ), ), ), ], ), SizedBox( width: 20, ), Image.asset( 'assets/images/menu.png', width: 30, height: 30, ) ], ) ], ); } }
Thank you :)